I quickly discovered that there are a tonne of default blocks in the Gutenberg editor, which is amazing.
In this lesson, I’ll go over a number of techniques for hiding or severely limiting the use of particular website blocks.
Gutenberg Block Manager
We won’t begin any code in this approach just yet. The Block Manager, a built-in function that enables you to turn on or off individual blocks on your website, was introduced in WordPress 5.2, making it possible to use this method. At the time this article is being written, the Block Manager can be found under Preferences > Blocks. Very cool, particularly if you are not a big coder.
Disable Gutenberg Blocks in Code
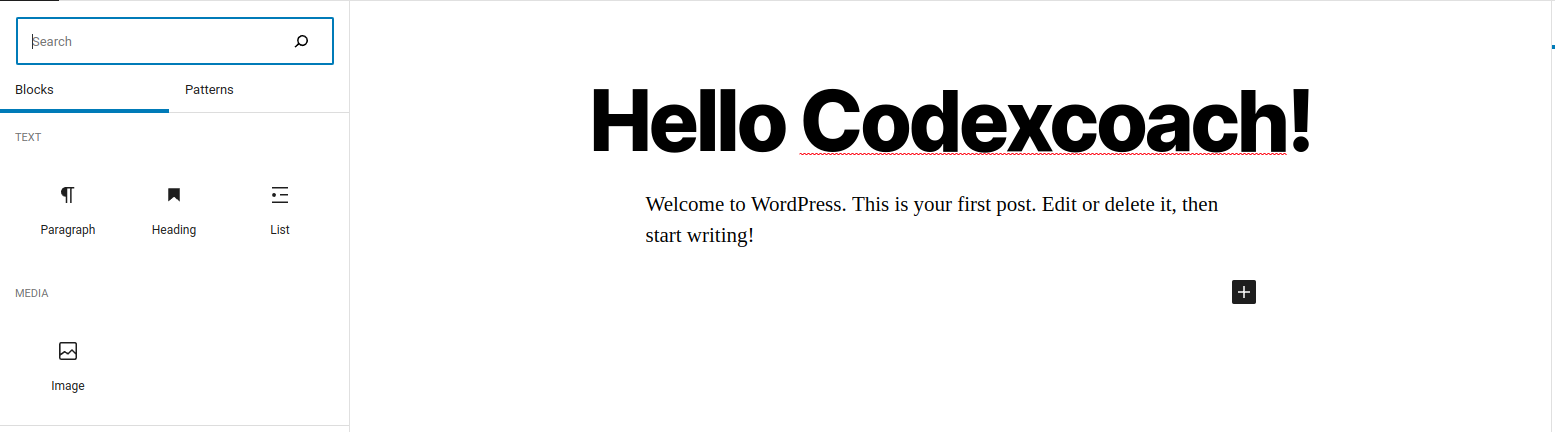
depending on your WordPress version, allowed block types We used to use allowed_block_types, but in WordPress 5.8 it was declared deprecated, therefore we now have to use the allowed_block_types_all filter.
Therefore, we can whitelist some specific blocks that we will allow to be used on the website by using the filter hook allowed_block_types_all. See the sample below, in which we will only show the paragraph, heading, image, and list blocks.
You would have this after adding this code to your current theme’s functions.php file.
<?php
add_filter( 'allowed_block_types_all', 'cxc_allowed_block_types_call_back', 25, 2 );
function cxc_allowed_block_types_call_back( $allowed_blocks, $editor_context ) {
return array(
'core/image',
'core/paragraph',
'core/heading',
'core/list',
'core/list-item',
'core/html'
);
}
?>
List of Gutenberg blocks editor
- List of Gutenberg blocks
Block slug | Block name |
---|---|
core/paragraph | Paragraph |
core/heading | Heading |
core/list and core/list-item | List |
core/preformatted | Preformatted |
core/pullquote | Pullquote |
core/table | Table |
core/verse | Verse |
- Media category
Block slug | Block name |
---|---|
core/image | Image |
core/gallery | Gallery |
core/audio | Audio |
core/cover | Cover |
core/file | File |
core/media-text | Media & Text |
core/video | Video |
- Design category
Block slug | Block name |
---|---|
core/buttons | Buttons |
core/columns | Columns |
core/group | Group |
core/row | Row |
core/stack | Stack |
core/more | More |
core/nextpage | Page Break |
core/separator | Separator |
core/spacer | Spacer |
- Widgets category
Block slug | Block name |
---|---|
core/archives | Archive |
core/calendar | Calendar |
core/categories | Categories |
core/html | Custom HTML |
core/latest-comments | Latest Comments |
core/latest-posts | Latest Posts |
core/page-list | Page List |
core/rss | RSS |
core/search | Search |
core/shortcode | Shortcode |
core/social-links | Social Icons |
core/tag-cloud | Tag Cloud |
- Theme category
Block slug | Block name |
---|---|
core/navigation | Navigation |
core/site-logo | Site Logo |
core/site-title | Site Title |
core/site-tagline | Site Tagline |
core/query | Query Loop |
core/posts-list | Posts List |
core/avatar | Avatar |
core/post-title | Post Title |
core/post-excerpt | Post Excerpt |
core/post-featured-image | Post Featured Image |
core/post-content | Post Content |
core/post-author | Post Author |
core/post-date | Post Date |
core/post-terms | Post Categories, Post Tags |
core/post-navigation-link | Next post, Previous post |
core/read-more | Read More |
core/comments-query-loop | Comments Query Loop |
core/post-comments-form | Post Comments Form |
core/loginout | Login/out |
core/term-description | Term Description |
core/query-title | Archive Title |
core/post-author-biography | Post Author Biography |
- Embeds category
Block slug | Block name |
---|---|
core/embed | Embed, Twitter, Youtube, WordPress, Soundcloud, Spotify, Flickr, Vimeo, Animoto, Cloudup, Crowdsignal, Dailymotion, Imgur, Issuu, Kickstarter, Mixcloud, Reddit, ReverbNation, Screencast, Scribd, Slideshare, SmugMug, Speaker Desc, TikTok, Videopress, WordPress.tv, Amazon Kindle, Pinterest, Wolfram |
Allow or Disallow Specific Post Type Blocks
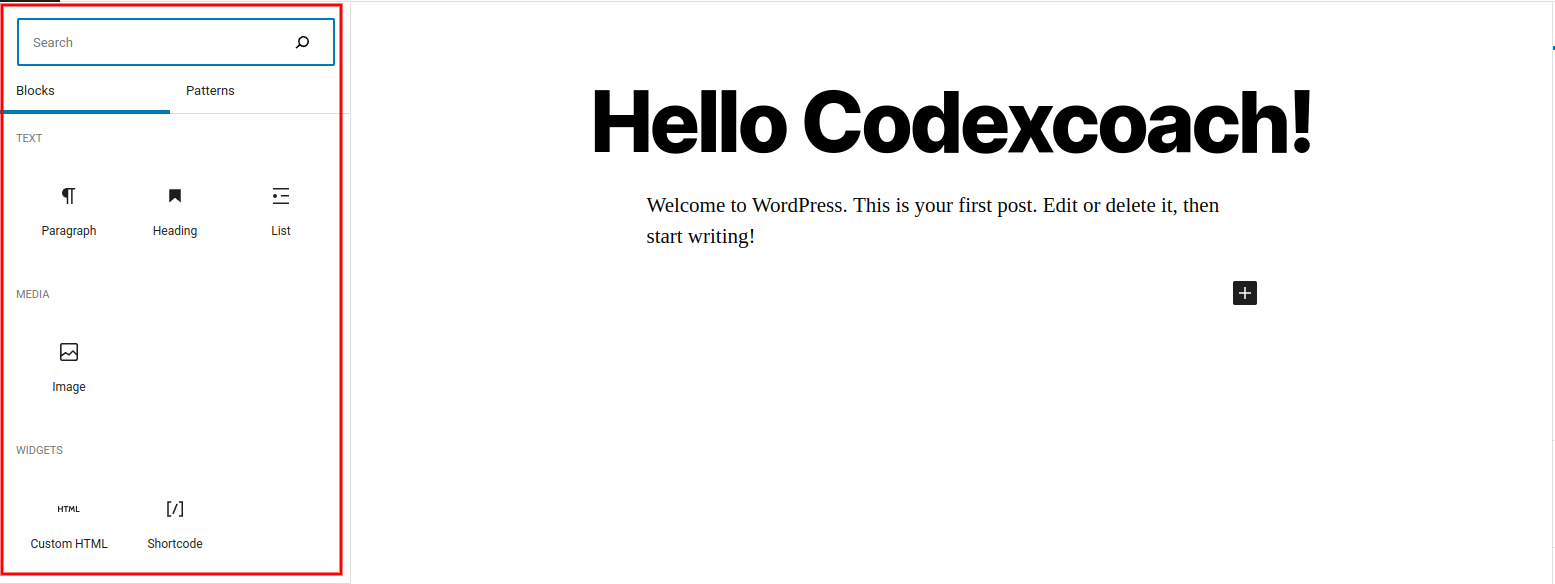
Do you recall that I stated the $editor_context option that the allowed_block_types_all hook accepts? Use it now, please! As an illustration, we’ll add a block called Shortcode for Posts. In this instance, a small adjustment will be made to our code.
<?php
add_filter( 'allowed_block_types_all', 'cxc_allowed_block_types_to_post_call_back', 25, 2 );
function cxc_allowed_block_types_to_post_call_back( $allowed_blocks, $editor_context ) {
$allowed_blocks = array(
'core/image',
'core/paragraph',
'core/heading',
'core/list',
'core/list-item',
'core/html'
);
// Show In Post Pages.
if( 'post' === $editor_context->post->post_type ) {
$allowed_blocks[] = 'core/shortcode';
}
return $allowed_blocks;
}
?>
This code sample retrieves the post type from a WP_Post object, which is found in $editor_context->post, as you probably already figured. Therefore, you can even permit or prohibit a certain block based on the post ID!
How to Blacklist Specific Blocks?
With the same allowed_block_types_all, we may blacklist any block, but we also require a list of all the registered blocks.
WP_Block_Type_Registry::get_instance()->get_all_registered()
will help us with that.
<?php
$registered_block_slugs = array_keys( WP_Block_Type_Registry::get_instance()->get_all_registered() );
echo '<pre>' . print_r( $registered_block_slugs, true ) . '</pre>';
/*
Array
(
[0] => core/archives
[1] => core/avatar
[2] => core/block
[3] => core/calendar
[4] => core/categories
... and so on
*/
?>
Okay, suppose you don’t require the Archives and Calendar blocks.
<?php
add_filter( 'allowed_block_types_all', 'cxc_blacklist_blocks_call_back' );
function cxc_blacklist_blocks_call_back( $allowed_blocks ) {
// get all the registered blocks
$blocks = WP_Block_Type_Registry::get_instance()->get_all_registered();
// then disable some of them
unset( $blocks[ 'core/archives' ] );
unset( $blocks[ 'core/calendar' ] );
// return the new list of allowed blocks
return array_keys( $blocks );
}
?>