WC()->cart->get_cart_contents_count() enables retrieval of a variety of cart items, together with a count of how many of each there are. This approach will yield 11 if your cart has 10 snowboards and 1 avocado toast, for instance! If you examine the source code for this method in WooCommerce, you can see it for yourself. It merely obtains the entire cart array from WC()->cart->get_cart() and then obtains the quantity parameter for each item.
This implementation of count(WC()->cart->get_cart()) really gives the number of distinct products, one for each product, in the cart.
Cart Total: <?php echo WC()->cart->get_cart_contents_count() ?>
Cart Total: <?php echo count( WC()->cart->get_cart() ) ?>
WooCommerce number of items count in cart
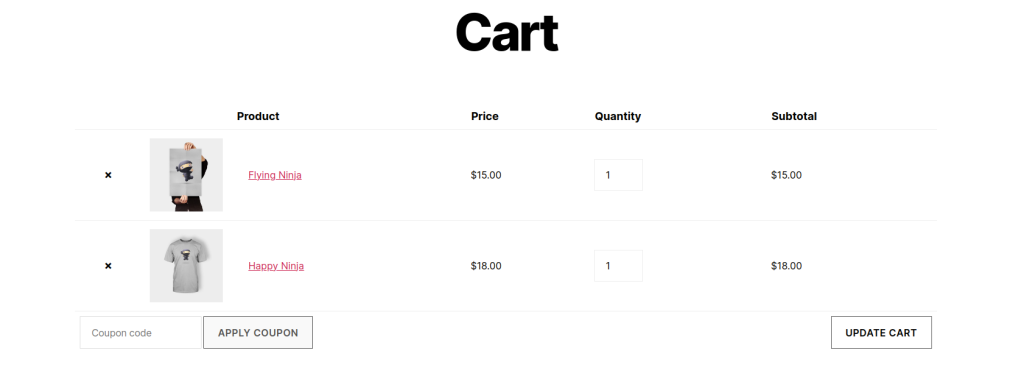
Getting the amount of products in your WooCommerce basket is a rather simple process.
<?php
global $woocommerce;
echo 'Cart Total: ' . $woocommerce->cart->get_cart_contents_count();
?>
It gives back the quantity of products in the WooCommerce cart. Following the code will also return the total number of goods in the cart, but it will only return unique items.
<?php
global $woocommerce;
echo 'Cart Total: ' . count( $woocommerce->cart->get_cart() );
?>
Now, let’s look at the output using both functions. Take note that the quantity is there in the screenshot.
<?php
// echo $woocommerce->cart->cart_contents_count;
Output : 2
// echo count( WC()->cart->get_cart() );
Output : 2
?>
Count every product just once using get_cart_contents_count()
What to do if your WooCommerce theme is already finished, get_cart_contents_count() is widely utilised, and you don’t want 100 products to appear in the cart count when customers have just added 100 of the same chocolate.
<?php
add_filter( 'woocommerce_cart_contents_count', 'cxc_get_cart_count_call_back' );
function cxc_get_cart_count_call_back( $count ) {
return count( WC()->cart->get_cart() );
}
?>
Excluding a certain product out of the cart count
<?php
add_filter( 'woocommerce_cart_contents_count', 'cxc_get_cart_count_excluding_product_call_back' );
function cxc_get_cart_count_excluding_product_call_back( $count ) {
//return count( WC()->cart->get_cart() );
$count = 0;
if( WC()->cart->get_cart() ){
foreach ( WC()->cart->get_cart() as $cart_item_key => $cart_item ) {
// just skip the loop iteration if it is a specific product_id
if( 181 === $cart_item[ 'product_id' ] ) {
continue;
}
$count++;
}
}
echo $count;
}
?>
When a product is added to the cart, the cart count is updated
When a product is added to the cart using Ajax, the cart count, budget, or cart menu that was previously inserted should be updated.
To begin, enable the WooCommerce Ajax add to cart setting. To access the option below, navigate to WooCommerce > Settings > Products. Just verified and saved it.
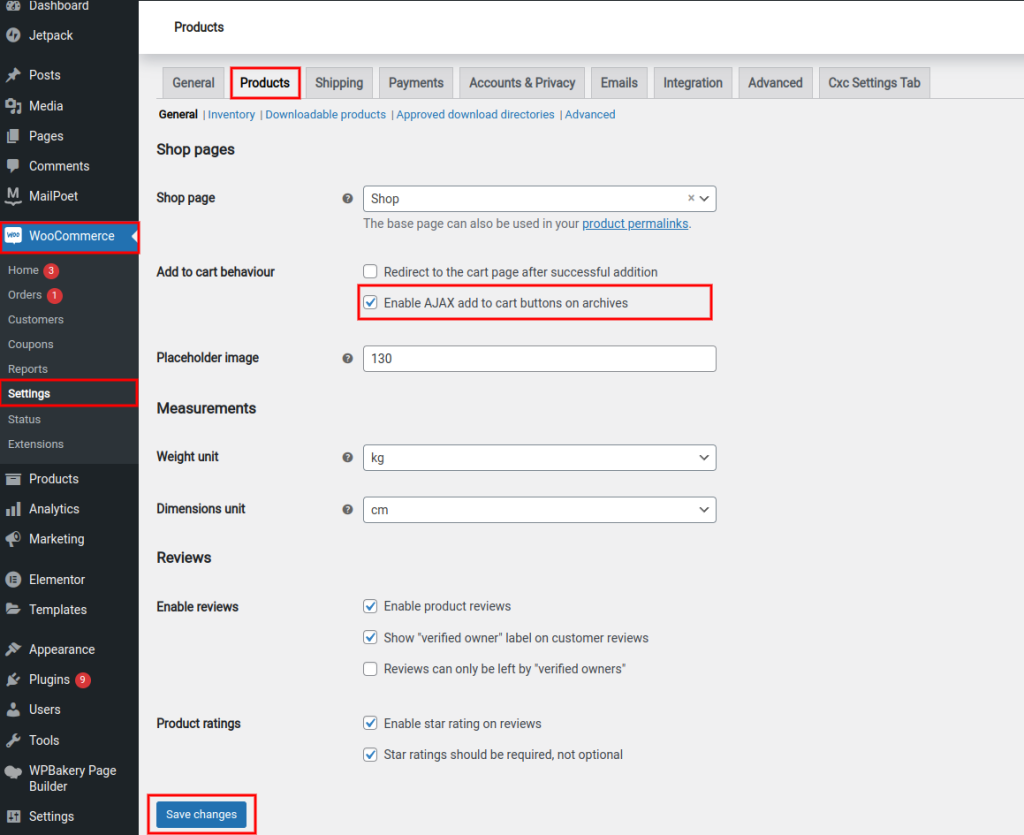
When you allow it, Ajax will be used to add the product to your basket. Therefore, the product will be added to your cart without a page refresh. Therefore, when a product is added to the cart using Ajax, we need to update the cart count number.
With the help of cart fragments, notably the extraordinarily useful hook woocommerce_add_to_cart_fragments, WooCommerce provides a mechanism for us to handle it.
<a class="cxc-cart" href="<?php echo wc_get_cart_url(); ?>" title="<?php _e( 'View your shopping cart' ); ?>"><?php echo sprintf ( _n( '%d item', '%d items', WC()->cart->get_cart_contents_count() ), WC()->cart->get_cart_contents_count() ); ?> – <?php echo WC()->cart->get_cart_total(); ?></a>
Let’s now look at how we can change the number of things in the cart when a new item is added.
Find the functions.php file from the theme that is currently active first. If it’s a parent theme, I advise making a child theme and making the necessary changes. This hook can also be used inside the main file for your plugin.
<?php
add_filter( 'woocommerce_add_to_cart_fragments', 'cxc_woocommerce_header_add_to_cart_fragment' );
function cxc_woocommerce_header_add_to_cart_fragment( $fragments ) {
global $woocommerce;
ob_start();
?>
<a class="cxc-cart" href="<?php echo esc_url(wc_get_cart_url()); ?>" title="<?php _e('View your shopping cart', 'woothemes'); ?>"><?php echo sprintf(_n('%d item', '%d items', $woocommerce->cart->cart_contents_count, 'woothemes'), $woocommerce->cart->cart_contents_count);?> – <?php echo $woocommerce->cart->get_cart_total(); ?></a>
<?php
$fragments['a.cxc-cart'] = ob_get_clean();
return $fragments;
}
?>