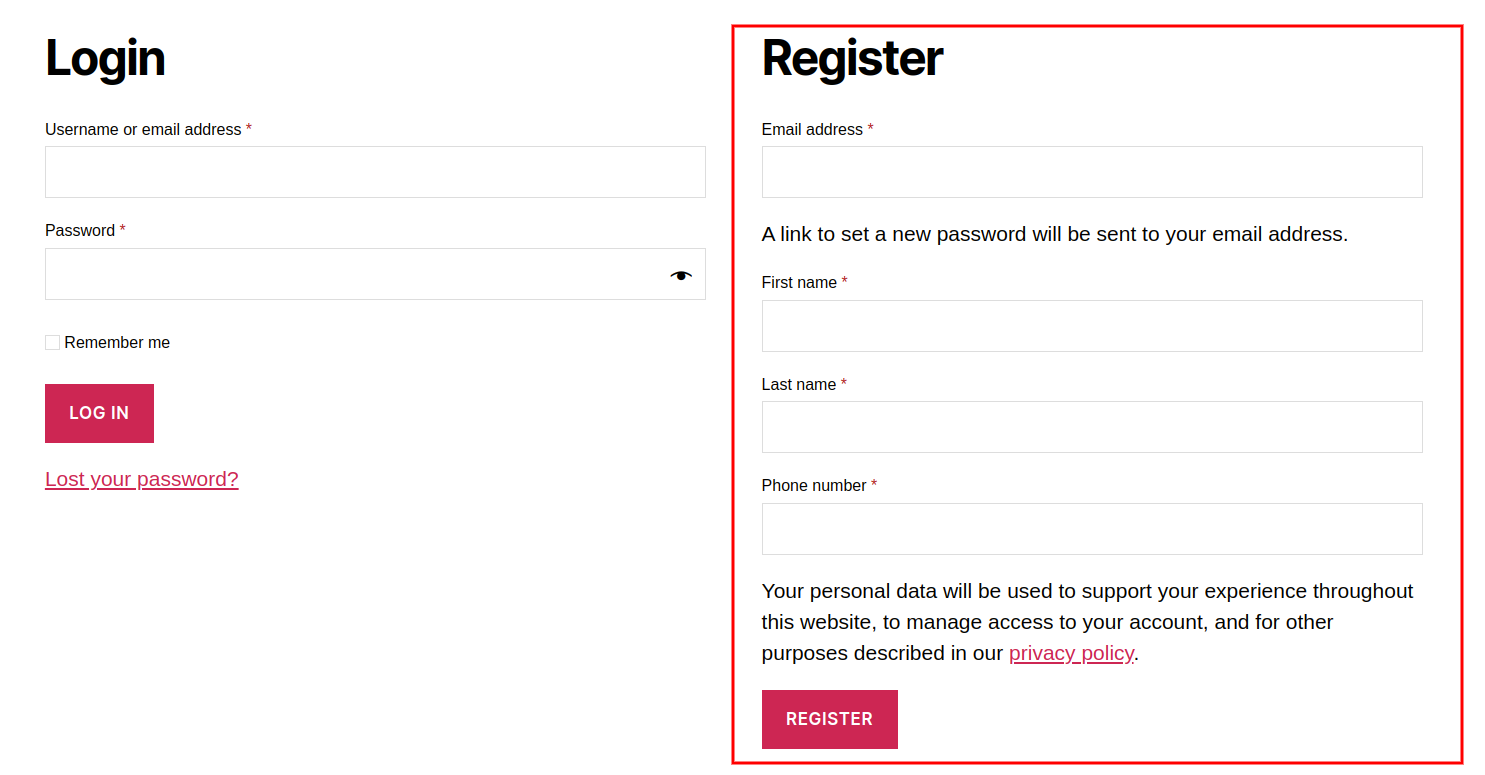
Your website will look more professional thanks to a custom form, which can also require extra user data throughout the registration process.
Enable Customer Registration Option
The account login page’s WooCommerce registration forms must first be activated. Check Enable Customer Registration on the “My Account” tab by going to WooCommerce → Settings → Accounts & Privacy and selecting that option.
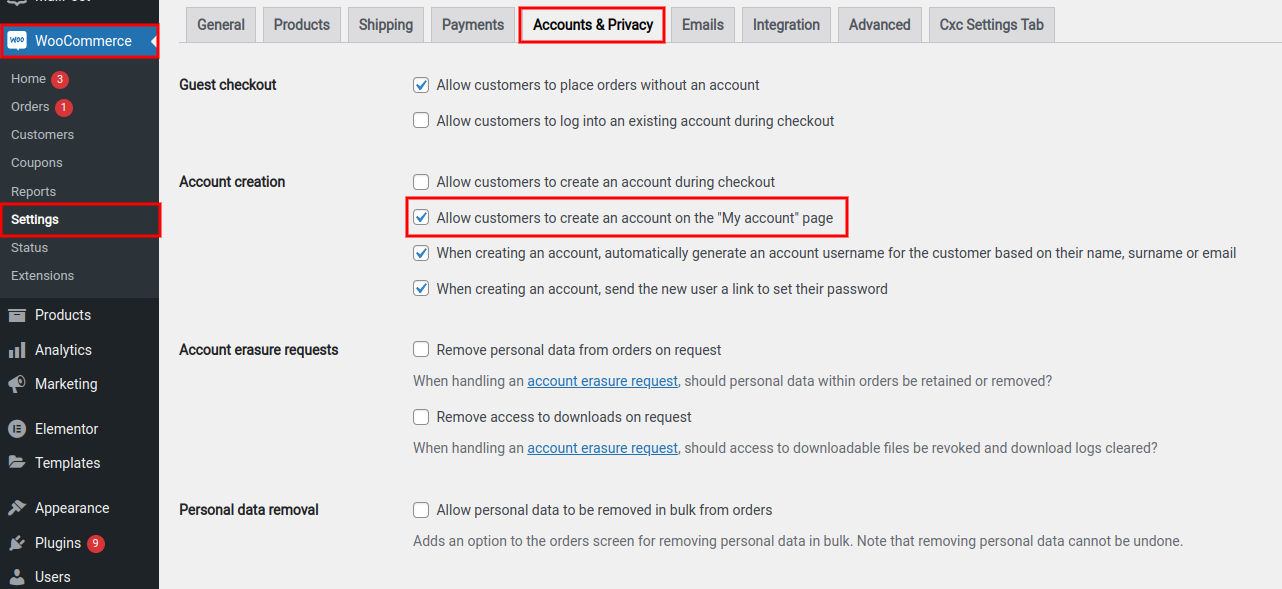
Add Custom Field in Functions.php File
It should be clear that it is a rather basic WooCommerce form. However, by using the subsequent operations, we can expand this structure with more fields. Include the following lines of code near the end of your functions.php file, which is found in your theme folder, to add more fields like first name, last name, phone number, etc.
<?php
// Cxc Add Custom Fields
add_action( 'woocommerce_register_form', 'cxc_add_register_form_field' );
function cxc_add_register_form_field(){
woocommerce_form_field(
'billing_first_name',
array(
'type' => 'text',
'required' => true, // just adds an "*"
'label' => 'First name'
),
( isset($_POST['billing_first_name']) ? $_POST['billing_first_name'] : '' )
);
woocommerce_form_field(
'billing_last_name',
array(
'type' => 'text',
'required' => true, // just adds an "*"
'label' => 'Last name'
),
( isset($_POST['billing_last_name']) ? $_POST['billing_last_name'] : '' )
);
woocommerce_form_field(
'billing_phone',
array(
'type' => 'tel',
'required' => true, // just adds an "*"
'label' => 'Phone number'
),
( isset( $_POST[ 'billing_phone' ] ) ? $_POST[ 'billing_phone' ] : '' )
);
}
?>
Validate Registration Form Fields
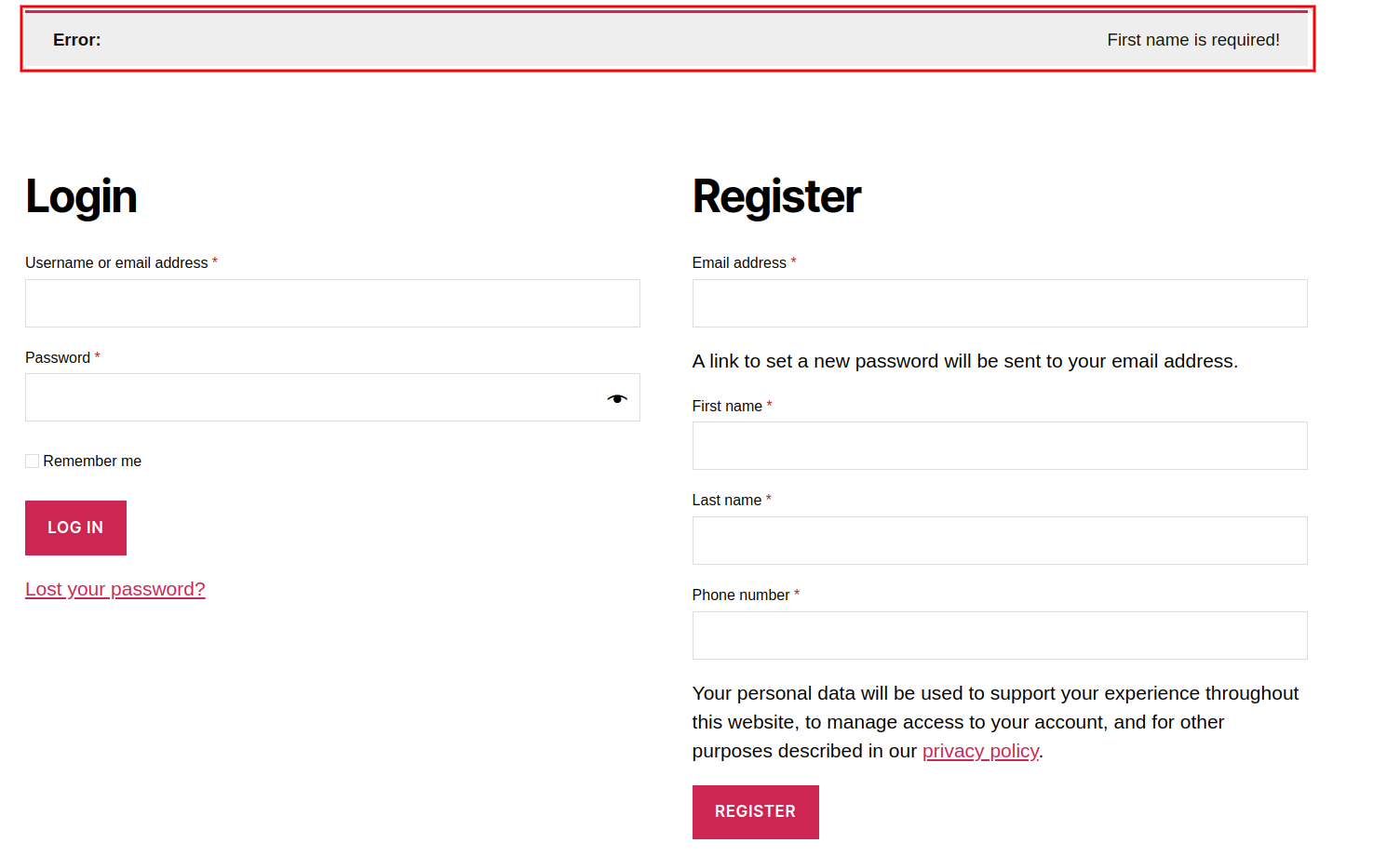
These recently added form fields now need to be validated as well. Include the preceding lines of code toward the end of your functions.php file, which is placed in the theme folder, to validate these structure fields.
<?php
// Cxc validate fields to Register Form
add_action( 'woocommerce_register_post', 'cxc_validate_fields', 10, 3 );
function cxc_validate_fields( $username, $email, $errors ) {
if ( empty( $_POST['billing_first_name'] ) ) {
$errors->add( 'billing_first_name_error', 'First name is required!' );
}
if ( empty( $_POST['billing_last_name'] ) ) {
$errors->add( 'billing_last_name_error', 'Last name is required!' );
}
if( empty( $_POST[ 'billing_phone' ] ) ) {
$errors->add( 'phone_number_error', 'We really want to know!' );
}
}
?>
Save Registration Form Fields
<?php
// Cxc save fields to database
add_action( 'woocommerce_created_customer', 'cxc_save_register_fields' );
function cxc_save_register_fields( $customer_id ){
if ( isset( $_POST['billing_first_name'] ) ) {
//First name field which is by default
update_user_meta( $customer_id, 'first_name', sanitize_text_field( $_POST['billing_first_name'] ) );
// First name field which is used in WooCommerce
update_user_meta( $customer_id, 'billing_first_name', sanitize_text_field( $_POST['billing_first_name'] ) );
}
if ( isset( $_POST['billing_last_name'] ) ) {
// Last name field which is by default
update_user_meta( $customer_id, 'last_name', sanitize_text_field( $_POST['billing_last_name'] ) );
// Last name field which is used in WooCommerce
update_user_meta( $customer_id, 'billing_last_name', sanitize_text_field( $_POST['billing_last_name'] ) );
}
if ( isset( $_POST[ 'billing_phone' ] ) ) {
update_user_meta( $customer_id, 'billing_phone', sanitize_text_field( $_POST[ 'billing_phone' ] ) );
}
}
?>