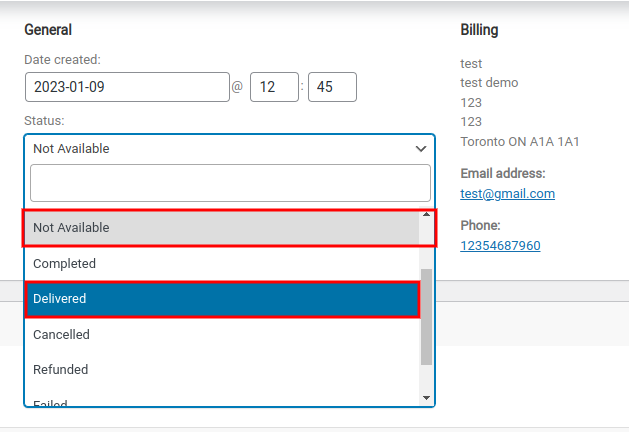
Register a custom order status
<?php
add_action( 'init', 'cxc_register_not_available_shipping_status' );
function cxc_register_not_available_shipping_status() {
register_post_status(
'wc-cxc-not-available',
array(
'label' => 'Not Available',
'public' => true,
'show_in_admin_status_list' => true,
'label_count' => _n_noop( 'Not Available (%s)', 'Not Available (%s)' )
)
);
register_post_status(
'wc-cxc-delivered',
array(
'label' => 'Delivered',
'public' => true,
'show_in_admin_status_list' => true,
'label_count' => _n_noop( 'Delivered (%s)', 'Delivered (%s)' )
)
);
}
?>
Add Register Status To List Of WC Order Status
<?php
add_filter( 'wc_order_statuses', 'cxc_register_add_status_to_list_fun' );
function cxc_register_add_status_to_list_fun( $order_statuses ) {
$new_status = array();
if( $order_statuses ){
foreach ( $order_statuses as $id => $label ) {
if ( 'wc-completed' === $id ) { // before "Completed" status
$new_status[ 'wc-cxc-not-available' ] = 'Not Available';
}
if ( 'wc-cancelled' === $id ) { // before "Cancelled" status
$new_status[ 'wc-cxc-delivered' ] = 'Delivered';
}
$new_status[ $id ] = $label;
}
}
return $new_status;
}
?>
Remove WC Order Status in WooCommerce
<?php
add_filter( 'wc_order_statuses', 'cxc_remove_register_status' );
function cxc_remove_register_status( $statuses ) {
// Remove Cancelled Order Status
if( isset( $statuses['wc-cancelled'] ) ){
unset( $statuses['wc-cancelled'] );
}
return $statuses;
}
?>
Change Order Status After Purchase
<?php
add_action( 'woocommerce_thankyou', 'cxc_change_aftre_order_status' );
function cxc_change_aftre_order_status( $order_id ) {
if ( ! $order_id ) {
return;
}
$order = wc_get_order( $order_id );
if( 'processing'== $order->get_status() ) {
$order->update_status( 'wc-on-hold' );
}
}
?>
Update Completed Order Status from a Returning Customer
<?php
add_action( 'woocommerce_thankyou', 'cxc_order_status_returning' );
function cxc_order_status_returning( $order_id ) {
// Get this customer orders
$user_id = wp_get_current_user();
$customer_orders = [];
if( wc_get_is_paid_statuses() ){
foreach ( wc_get_is_paid_statuses() as $paid_status ) {
$customer_orders += wc_get_orders( [
'type' => 'shop_order',
'limit' => - 1,
'customer_id' => $user_id->ID,
'status' => $paid_status,
] );
}
}
# previous order exists
if( count( $customer_orders ) > 0 ) {
if ( ! $order_id ) {
return;
}
$order = wc_get_order( $order_id );
if( 'processing'== $order->get_status() ) {
$order->update_status( 'wc-completed' );
}
}
}
?>
Was this article helpful?
YesNo