Checkout Reordering Fields within a Field Group
To reorder the WooCommerce checkout fields, you can use a number of code snippets on your website. But first, you should know that the fields are divided into four categories:
billing
– Billing Address,shipping
– Shipping Address,account
– Account Login,order
– Additional information.
Reorder Checkout fields priority
Name | Priority |
---|---|
billing_first_name | 10 |
billing_last_name | 20 |
billing_company | 30 |
billing_country | 40 |
billing_address_1 | 50 |
billing_address_2 | 60 |
billing_city | 70 |
billing_state | 80 |
billing_postcode | 90 |
billing_phone | 100 |
billing_email | 110 |
shipping_first_name | 10 |
shipping_last_name | 20 |
shipping_company | 30 |
shipping_country | 40 |
shipping_address_1 | 50 |
shipping_address_2 | 60 |
shipping_city | 70 |
shipping_state | 80 |
shipping_postcode | 90 |
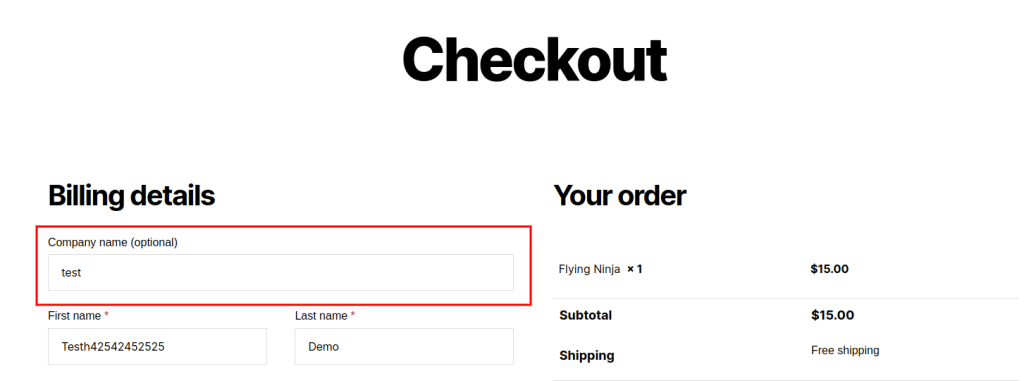
<?php
add_filter( 'woocommerce_checkout_fields', 'cxc_checkout_billing_company_first' );
function cxc_checkout_billing_company_first( $checkout_fields ) {
$checkout_fields[ 'billing' ][ 'billing_company' ][ 'priority' ] = 4;
return $checkout_fields;
}
?>
Actually, depending on its position, each checkout field has its own style (CSS class); there are three main classes:
form-row-first
– half-width, first,form-row-last
– half-width, last,form-row-wide
– fullwidth.
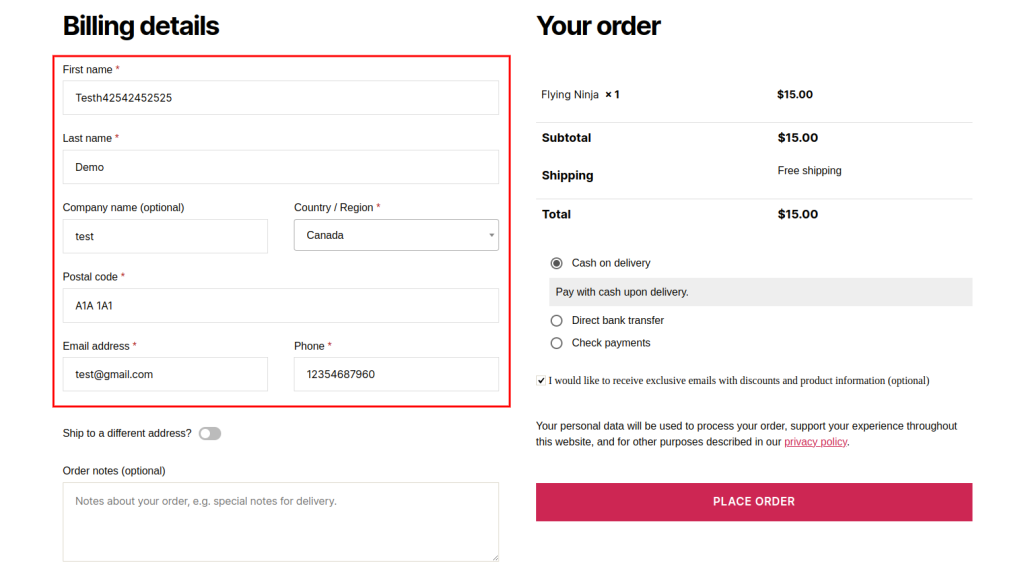
<?php
add_filter( 'woocommerce_checkout_fields' , 'cxc_checkout_billing_fields_styling', 99 );
function cxc_checkout_billing_fields_styling( $checkout_fields ) {
$checkout_fields['billing']['billing_first_name']['class'][0] = 'form-row-wide';
$checkout_fields['billing']['billing_last_name']['class'][0] = 'form-row-wide';
$checkout_fields['billing']['billing_company']['class'][0] = 'form-row-first';
$checkout_fields['billing']['billing_country']['class'][0] = 'form-row-last';
$checkout_fields['billing']['billing_email']['class'][0] = 'form-row-first';
$checkout_fields['billing']['billing_phone']['class'][0] = 'form-row-last';
return $checkout_fields;
}
?>
Phone Fields to Another Field Group
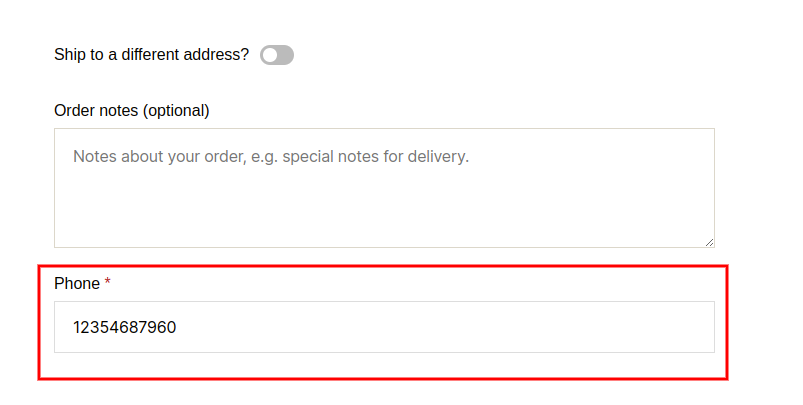
<?php
add_filter( 'woocommerce_checkout_fields', 'cxc_billing_phone_another_group' );
function cxc_billing_phone_another_group( $checkout_fields ){
// 1. We assign a field array to another group here
$checkout_fields[ 'order' ][ 'billing_phone' ] = $checkout_fields[ 'billing' ][ 'billing_phone' ];
// 2. Remove a field from a previous location
unset( $checkout_fields[ 'billing' ][ 'billing_phone' ] );
return $checkout_fields;
}
?>
Was this article helpful?
YesNo