Include an Attribute Type
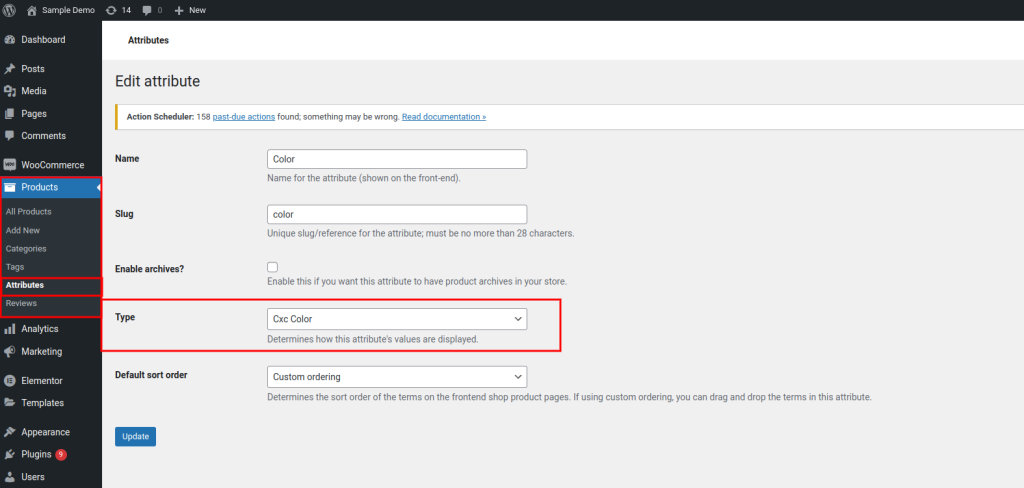
<?php
add_filter( 'product_attributes_type_selector', 'cxc_add_attr_color_type' );
function cxc_add_attr_color_type( $types ) {
$types[ 'cxc_color_type' ] = 'Cxc Color'; // "cxc_color_type" // is a custom slug
return $types;
}
?>
Register and enqueue a custom stylesheet in the WordPress admin.
<?php
add_action( 'admin_enqueue_scripts', 'cxc_admin_register_styles_script' );
function cxc_admin_register_styles_script(){
wp_enqueue_style( 'wp-color-picker');
wp_enqueue_script( 'wp-color-picker');
}
add_action( 'admin_footer', 'cxc_admin_add_custom_js_functions' );
function cxc_admin_add_custom_js_functions(){
?>
<script type="text/javascript">
jQuery( document ).ready( function() {
jQuery( '#term-cxc_color_type' ).wpColorPicker();
} );
</script>
<?php
}
?>
Make a Color Picker Field available in the Attribute Terms.
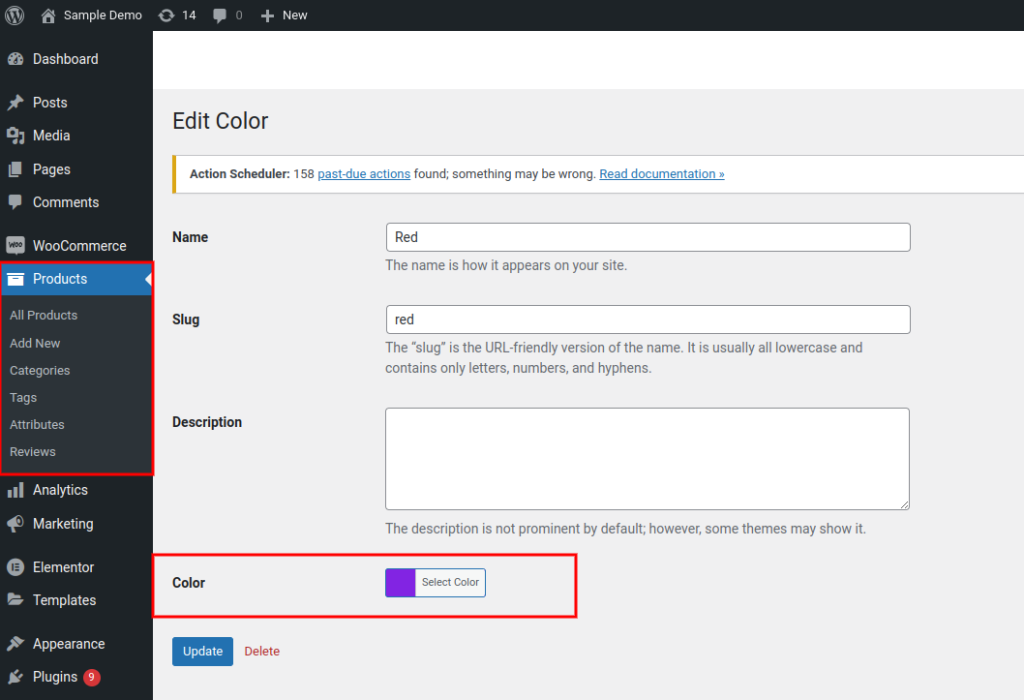
<?php
add_action( 'pa_color_edit_form_fields', 'cxc_color_edit_fields', 10, 2 );
function cxc_color_edit_fields( $term, $taxonomy ){
global $wpdb;
$attribute_type = $wpdb->get_var(
$wpdb->prepare(
"
SELECT attribute_type
FROM " . $wpdb->prefix . "woocommerce_attribute_taxonomies
WHERE attribute_name = '%s'
",
substr( $taxonomy, 3 ) // remove "pa_" prefix
)
);
if( 'cxc_color_type' !== $attribute_type ) {
return;
}
$color = get_term_meta( $term->term_id, 'cxc_color_type', true );
?>
<tr class="form-field">
<th><label for="term-cxc_color_type">Color</label></th>
<td><input type="text" id="term-cxc_color_type" name="cxc_color_type" value="<?php echo esc_attr( $color ) ?>" /></td>
</tr>
<?php
}
?>
Store the value of the field as term meta.
<?php
add_action( 'edited_pa_color', 'cxc_save_color_call_back' );
function cxc_save_color_call_back( $term_id ) {
$cxc_color_type = ! empty( $_POST[ 'cxc_color_type' ] ) ? $_POST[ 'cxc_color_type' ] : '';
update_term_meta( $term_id, 'cxc_color_type', sanitize_hex_color( $cxc_color_type ) );
}
?>
Do Attribute on the Edit Product Page
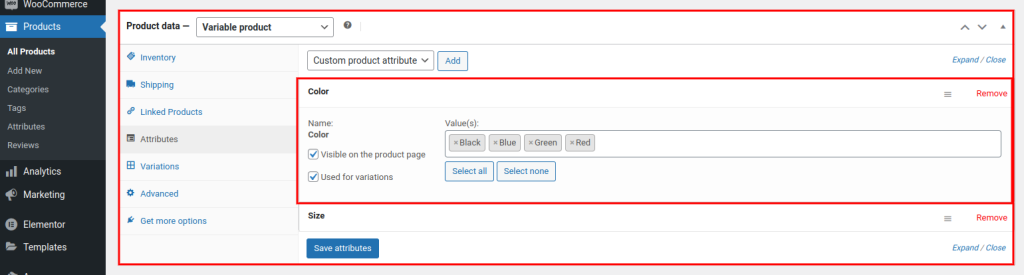
<?php
add_action( 'woocommerce_product_option_terms', 'cxc_color_attr_select_call_back', 10, 3 );
function cxc_color_attr_select_call_back( $attribute_taxonomy, $i, $attribute ) {
if( 'cxc_color_type' !== $attribute_taxonomy->attribute_type ) {
return;
}
$options = $attribute->get_options();
$options = ! empty( $options ) ? $options : array();
?>
<select multiple="multiple" data-placeholder="Select color" class="multiselect attribute_values wc-enhanced-select" name="attribute_values[<?php echo $i ?>][]">
<?php
$colors = get_terms( 'pa_color', array( 'hide_empty' => 0 ) );
if( $colors ) {
foreach ( $colors as $color ) {
echo '<option value="' . $color->term_id . '"' . wc_selected( $color->term_id, $options ) . '>' . $color->name . '</option>';
}
}
?>
</select>
<button class="button plus select_all_attributes">Select all</button>
<button class="button minus select_no_attributes">Select none</button>
<?php
}
?>
Finally Results :
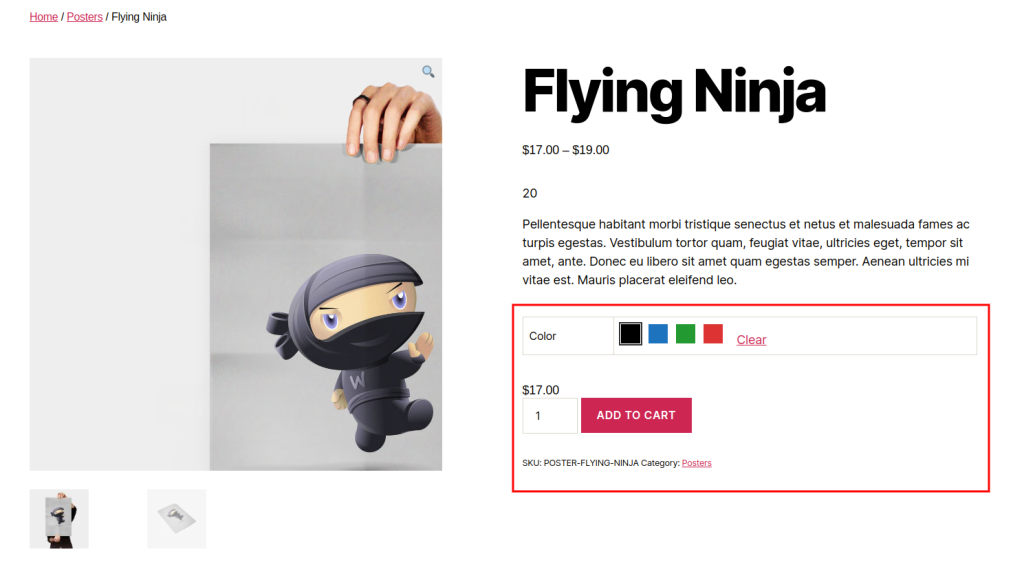
<?php
add_filter( 'woocommerce_dropdown_variation_attribute_options_html', 'cxc_color_swatches_html_call_back', 20, 2 );
function cxc_color_swatches_html_call_back( $html, $args ){
global $wpdb;
$taxonomy = $args[ 'attribute' ];
$product = $args[ 'product' ];
$attribute_type = $wpdb->get_var(
$wpdb->prepare(
"
SELECT attribute_type
FROM " . $wpdb->prefix . "woocommerce_attribute_taxonomies
WHERE attribute_name = '%s'
",
substr( $taxonomy, 3 )
)
);
if( 'cxc_color_type' !== $attribute_type ) {
return $html;
}
$html = '<div style="display:none">' . $html . '</div>';
$colors = wc_get_product_terms( $product->get_id(), $taxonomy );
foreach( $colors as $color ) {
if( in_array( $color->slug, $args[ 'options' ] ) ) {
$hex_color = get_term_meta( $color->term_id, 'cxc_color_type', true );
$selected = $args[ 'selected' ] === $color->slug ? 'color-selected' : '';
$html .= sprintf(
'<span class="cxc-color-swatch %s" style="background-color:%s;" title="%s" data-value="%s"></span>',
$selected,
$hex_color,
$color->name,
$color->slug
);
}
}
return $html;
}
?>
In your theme you can include css and js like this wp_head action.
<?php
add_action( 'wp_head', 'cxc_add_custom_style_script_functions' );
function cxc_add_custom_style_script_functions(){
?>
<style type="text/css">
.cxc-color-swatch {
width: 30px;
height: 30px;
display: inline-block;
margin-right: 10px;
cursor:pointer;
border: 1px solid #fff;
outline: 2px solid #fff;
}
.cxc-color-swatch.selected{
outline: 1px solid #333;
}
</style>
<script type="text/javascript">
jQuery( document ).ready( function() {
jQuery(document).on( 'click', '.variations_form .cxc-color-swatch', function ( e ) {
var cxc_this = jQuery( this );
var cxc_select = cxc_this.closest( '.value' ).find( 'select' );
var cxc_val = cxc_this.data( 'value' );
cxc_this.addClass( 'selected' ).siblings( '.selected' ).removeClass( 'selected' );
cxc_select.val( cxc_val );
cxc_select.change();
} );
} );
</script>
<?php
}
?>
Was this article helpful?
YesNo