Have you ever gotten bored with WordPress’s default login page? Yes, I’m talking about that plain and dull page where you just have to enter your credentials every single time. If you’re using a custom design theme, the login page could be one of the reasons for your frustration.
Note: If the action code will not work, then change action wp to init.
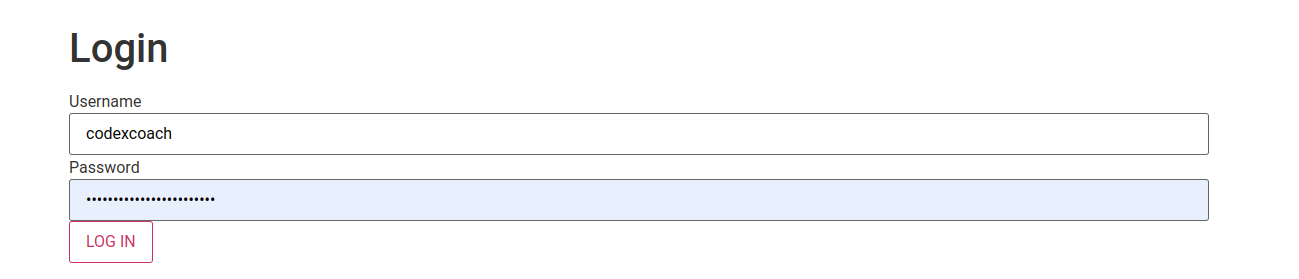
Login Form | Shortcode: [cxc_login_form]
<?php
add_shortcode( 'cxc_login_form', 'cxc_login_form_callback' );
function cxc_login_form_callback() {
ob_start();
if ( !is_user_logged_in() ) {
global $errors_login;
if (!empty( $errors_login ) ) {
?>
<div class="alert alert-danger">
<?php echo $errors_login; ?>
</div>
<?php } ?>
<form method="post" class="wc-login-form">
<div class="login_form">
<div class="log_user">
<label for="user_name">Username</label>
<input name="log" type="text" id="user_name" value="<?php echo isset($_POST['log']) ? $_POST['log'] : ''; ?>">
</div>
<div class="log_pass">
<label for="user_password">Password</label>
<input name="pwd" id="user_password" type="password">
</div>
<?php
ob_start();
do_action( 'login_form' );
echo ob_get_clean();
?>
<?php wp_nonce_field( 'userLogin', 'formType' ); ?>
</div>
<button type="submit">LOG IN</button>
</form>
<?php
} else {
echo '<p class="error-logged">You are already logged in.</p>';
}
$login_form = ob_get_clean();
return $login_form;
}
add_action( 'wp', 'cxc_user_login_callback' );
function cxc_user_login_callback() {
if ( isset( $_POST['formType'] ) && wp_verify_nonce( $_POST['formType'], 'userLogin' ) ) {
global $errors_login;
$uName = $_POST['log'];
$uPassword = $_POST['pwd'];
if ($uName == '' && $uPassword != '') {
$errors_login = '<strong>Error! </strong> Username is required.';
} elseif ($uName != '' && $uPassword == '') {
$errors_login = '<strong>Error! </strong> Password is required.';
} elseif ($uName == '' && $uPassword == '') {
$errors_login = '<strong>Error! </strong> Username & Password are required.';
} elseif ($uName != '' && $uPassword != '') {
$creds = array();
$creds['user_login'] = $uName;
$creds['user_password'] = $uPassword;
$creds['remember'] = false;
$user = wp_signon( $creds, false );
if ( is_wp_error($user) ) {
$errors_login = $user->get_error_message();
} else {
wp_set_current_user( $user->ID );
wp_set_auth_cookie( $user->ID );
do_action( 'wp_login', $user->user_login, $user );
wp_redirect( site_url() );
exit;
}
}
}
}
?>
For more details please visit this post.
Register Form | Shortcode: [cxc_register_form]
Do you want to create a custom registration or sign-up form in WordPress without using a single plugin? Then this is the perfect place for you.
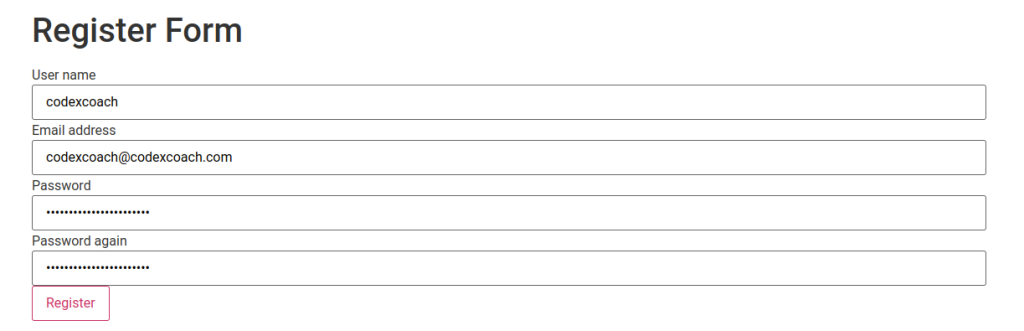
Copy the code below and paste it into your function.php file.
<?php
add_shortcode( 'cxc_register_form', 'cxc_register_form_callback' );
function cxc_register_form_callback() {
ob_start();
if ( !is_user_logged_in() ) {
global $registrationError, $registrationSuccess;
if ( !empty( $registrationError ) ) {
?>
<div class="alert alert-danger">
<?php echo $registrationError; ?>
</div>
<?php } ?>
<?php if ( !empty( $registrationSuccess ) ) { ?>
<br/>
<div class="alert alert-success">
<?php echo $registrationSuccess; ?>
</div>
<?php } ?>
<form method="post" class="wc-register-form">
<div class="register_form">
<div class="log_user">
<label for="user_name">User name</label>
<?php $user_name = isset($_POST['user_name']) ? $_POST['user_name'] : ''; ?>
<input type="text" name="user_name" id="user_name" value="<?php echo $user_name; ?>" />
</div>
<div class="log_user">
<label for="user_email">Email address</label>
<?php $user_email = isset($_POST['user_email']) ? $_POST['user_email'] : ''; ?>
<input type="email" name="user_email" id="user_email" value="<?php echo $user_email; ?>" />
</div>
<div class="log_pass">
<label for="user_password">Password</label>
<input type="password" name="user_password" id="user_password" />
</div>
<div class="log_pass">
<label for="user_cpassword">Password again</label>
<input type="password" name="user_cpassword" id="user_cpassword" />
</div>
<div class="log_pass">
<?php
ob_start();
do_action( 'register_form' );
echo ob_get_clean();
?>
</div>
<div class="log_user">
<?php wp_nonce_field( 'userRegister', 'formType' ); ?>
<button type="submit" class="register_user">Register</button>
</div>
</div>
</form>
<?php
} else {
echo '<p class="error-logged">You are already logged in.</p>';
}
$register_form = ob_get_clean();
return $register_form;
}
add_action( 'wp', 'cxc_user_register_callback' );
function cxc_user_register_callback() {
if ( isset( $_POST['formType'] ) && wp_verify_nonce( $_POST['formType'], 'userRegister') ) {
global $registrationError, $registrationSuccess;
$u_name = trim( $_POST['user_name'] );
$u_email = trim( $_POST['user_email'] );
$u_pwd = trim( $_POST['user_password'] );
$u_cpwd = trim( $_POST['user_cpassword'] );
if ( $u_name == '' ) {
$registrationError .= '<strong>Error! </strong> Enter User name.,';
}
if ( username_exists( $u_name ) ) {
$registrationError .= '<strong>Error! </strong> Username In Use!.,';
}
if ( $u_email == '' ) {
$registrationError .= '<strong>Error! </strong> Enter Email.,';
}
if ( $u_pwd == '' || $u_cpwd == '' ) {
$registrationError .= '<strong>Error! </strong> Enter Password.,';
}
if ( strlen( $u_pwd ) < 7) {
$registrationError .= '<strong>Error! </strong> Use minimum 7 character in password.,';
}
if ( $u_pwd != $u_cpwd ) {
$registrationError .= '<strong>Error! </strong> Password are not matching.,';
}
if ( $u_email != '' && !is_email( $u_email ) ) {
$registrationError .= '<strong>Error! </strong> Invalid e-mail address.,';
}
if ( email_exists( $u_email ) != false ) {
$registrationError .= '<strong>Error! </strong> This Email is already registered.,';
}
$registrationError = trim( $registrationError, ',' );
$registrationError = str_replace( ",", "<br/>", $registrationError );
if ( empty( $registrationError ) ) {
$user_login = $u_name;
$user_email = $u_email;
$userdata = array(
'user_login' => $user_login,
'user_pass' => $u_pwd,
'user_email' => $user_email
);
$user_id = wp_insert_user( $userdata );
if ( is_wp_error( $user ) ) {
$registrationError = $user->get_error_message();
} else {
$registrationSuccess = '<strong>Success! </strong> Application submitted. Please wait for user approval.';
$user = get_userdata( $user_id );
wp_set_current_user( $user->ID );
wp_set_auth_cookie( $user->ID );
do_action( 'wp_login', $user->user_login, $user );
wp_redirect( site_url() );
exit;
}
}
}
}
?>
Click here to know more.
Change Password Form | Shortcode: [cxc_change_pwd_form]
Imagine your users logging in to your website and immediately seeing the login interface. How about the user interface for changing their password?
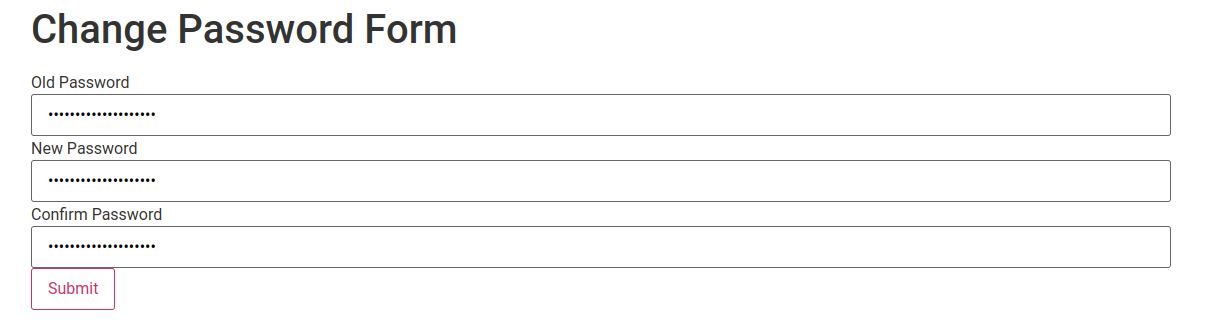
Copy the code below and paste it into your function.php file.
<?php
add_shortcode( 'cxc_change_pwd_form', 'cxc_change_pwd_form_callback' );
function cxc_change_pwd_form_callback() {
ob_start();
if ( is_user_logged_in() ) {
global $changePasswordError, $changePasswordSuccess;
if ( !empty( $changePasswordError ) ) {
?>
<div class="alert alert-danger">
<?php echo $changePasswordError; ?>
</div>
<?php } ?>
<?php if ( !empty( $changePasswordSuccess ) ) { ?>
<br/>
<div class="alert alert-success">
<?php echo $changePasswordSuccess; ?>
</div>
<?php } ?>
<form method="post" class="wc-change-pwd-form">
<div class="change_pwd_form">
<div class="log_pass">
<label for="user_oldpassword">Old Password</label>
<input type="password" name="user_opassword" id="user_oldpassword" />
</div>
<div class="log_pass">
<label for="user_password">New Password</label>
<input type="password" name="user_password" id="user_password" />
</div>
<div class="log_pass">
<label for="user_cpassword">Confirm Password</label>
<input type="password" name="user_cpassword" id="user_cpassword" />
</div>
<div class="log_pass">
<?php
ob_start();
do_action( 'password_reset' );
echo ob_get_clean();
?>
</div>
<div class="log_user">
<?php wp_nonce_field( 'changePassword', 'formType' ); ?>
<button type="submit" class="register_user">Submit</button>
</div>
</div>
</form>
<?php
}
$change_pwd_form = ob_get_clean();
return $change_pwd_form;
}
add_action( 'wp', 'cxc_user_change_pwd_callback' );
function cxc_user_change_pwd_callback() {
if ( isset( $_POST['formType'] ) && wp_verify_nonce( $_POST['formType'], 'changePassword' ) ) {
global $changePasswordError, $changePasswordSuccess;
$user = wp_get_current_user();
$changePasswordError = '';
$changePasswordSuccess = '';
$u_opwd = trim( $_POST['user_opassword'] );
$u_pwd = trim( $_POST['user_password'] );
$u_cpwd = trim( $_POST['user_cpassword'] );
if ( $u_opwd == '' || $u_pwd == '' || $u_cpwd == '' ) {
$changePasswordError .= '<strong>ERROR: </strong> Enter Password.,';
}
if ( !wp_check_password( $u_opwd, $user->data->user_pass, $user->ID ) ) {
$changePasswordError .= '<strong>ERROR: </strong> Old Password wrong.,';
}
if ( $u_pwd != $u_cpwd ) {
$changePasswordError .= '<strong>ERROR: </strong> Password are not matching.,';
}
if ( strlen( $u_pwd ) < 7 ) {
$changePasswordError .= '<strong>ERROR: </strong> Use minimum 7 character in password.,';
}
$changePasswordError = trim( $changePasswordError, ',' );
$changePasswordError = str_replace( ",", "<br/>", $changePasswordError );
if ( empty( $changePasswordError ) ) {
wp_set_password( $u_pwd, $user->ID );
do_action( 'wp_login', $user->user_login, $user );
wp_set_current_user( $user->ID );
wp_set_auth_cookie( $user->ID );
$changePasswordSuccess = 'Password is successfully updated.';
}
}
}
?>
For more details visit here: WordPress Change Password page
Forgot Password Form | Shortcode: [cxc_forgot_pwd_form]
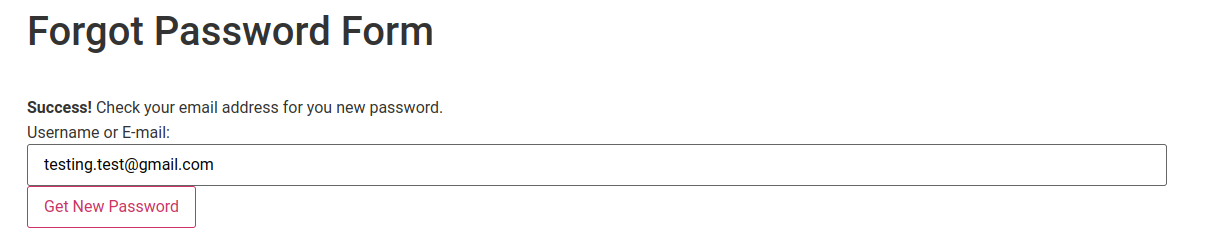
Create a forgot password form without using a WordPress plugin.
<?php
add_shortcode( 'cxc_forgot_pwd_form', 'cxc_forgot_pwd_form_callback' );
function cxc_forgot_pwd_form_callback() {
ob_start();
if ( !is_user_logged_in() ) {
global $getPasswordError, $getPasswordSuccess;
if ( !empty( $getPasswordError ) ) {
?>
<div class="alert alert-danger">
<?php echo $getPasswordError; ?>
</div>
<?php } ?>
<?php if ( !empty( $getPasswordSuccess ) ) { ?>
<br/>
<div class="alert alert-success">
<?php echo $getPasswordSuccess; ?>
</div>
<?php } ?>
<form method="post" class="wc-forgot-pwd-form">
<div class="forgot_pwd_form">
<div class="log_user">
<label for="user_login">Username or E-mail:</label>
<?php $user_login = isset($_POST['user_login']) ? $_POST['user_login'] : ''; ?>
<input type="text" name="user_login" id="user_login" value="<?php echo $user_login; ?>" />
</div>
<div class="log_user">
<?php
ob_start();
do_action( 'lostpassword_form' );
echo ob_get_clean();
?>
<?php wp_nonce_field('userGetPassword', 'formType'); ?>
<button type="submit" class="get_new_password">Get New Password</button>
</div>
</div>
</form>
<?php
}
$forgot_pwd_form = ob_get_clean();
return $forgot_pwd_form;
}
add_action('wp', 'cxc_user_forgot_pwd_callback');
function cxc_user_forgot_pwd_callback() {
if ( isset( $_POST['formType'] ) && wp_verify_nonce( $_POST['formType'], 'userGetPassword' ) ) {
global $getPasswordError, $getPasswordSuccess;
$email = trim( $_POST['user_login'] );
if ( empty( $email ) ) {
$getPasswordError = '<strong>Error! </strong>Enter a e-mail address.';
} else if ( !is_email( $email ) ) {
$getPasswordError = '<strong>Error! </strong>Invalid e-mail address.';
} else if ( !email_exists( $email ) ) {
$getPasswordError = '<strong>Error! </strong>There is no user registered with that email address.';
} else {
// lets generate our new password
$random_password = wp_generate_password( 12, false );
// Get user data by field and data, other field are ID, slug, slug and login
$user = get_user_by( 'email', $email );
$update_user = wp_update_user( array(
'ID' => $user->ID,
'user_pass' => $random_password
) );
// if update user return true then lets send user an email containing the new password
if ( $update_user ) {
$to = $email;
$subject = 'Your new password';
$sender = get_bloginfo( 'name' );
$message = 'Your new password is: ' . $random_password;
/* $headers[] = 'MIME-Version: 1.0' . "\r\n";
$headers[] = 'Content-type: text/html; charset=iso-8859-1' . "\r\n";
$headers[] = "X-Mailer: PHP \r\n";
$headers[] = 'From: ' . $sender . ' < ' . $email . '>' . "\r\n"; */
$headers = array( 'Content-Type: text/html; charset=UTF-8' );
$mail = wp_mail( $to, $subject, $message, $headers );
if ( $mail ) {
$getPasswordSuccess = '<strong>Success! </strong>Check your email address for you new password.';
}
} else {
$getPasswordError = '<strong>Error! </strong>Oops something went wrong.';
}
}
}
}
?>
Get more details from here.
I using you code in localhost. But Not showing anything in front-end if i use you code in functions.php and add short code in template. I have also used init instead wp but nothing showing anything. I also checked any one can register from admin but no any response. Is there any problem in this code
How you added shortcodes in templates file? Hard coded shortcode will work. You have to write
do_shortcode('[wc_login_form]');
on template file.any similar code to modify email?
Thank you very much for this article. It’s great!
It works perfectly. Now I would like to restrict registration by email domain in this code. I have added this in callback function in wc-register-form.php:
$d_mail = substr(strrchr($u_email, “@”), 1); // Gets the domain in the form
$allowed_domain = “mydomain.com”; // Allowed domain
if ($d_email !== $allowed_domain) {
$registrationError .= ‘Error! You must enter an email domain mydomain.com.,’;
}
This works the first time but it seems like $registrationError stays on even though I enter a correct email.
Please, tell me how can I correct my mistake.
I have already solved it. It was a very silly mistake. $d_email was not defined it was $d_mail.
Thank you very much again.
Wonderfull bro
have you any idea about logout?
Yes sure…please contact us